3 Getting Started - Reference Documentation
Authors: Marc Palmer (marc@grailsrocks.com)
Version: 1.0.RC2
Table of Contents
3 Getting Started
This section will take you through the process of creating a simple application that shows you how the Theme and UI APIs work together to provide pluggable UI rendering.To get started you need to install the Platform UI plugin, and for this example you'll also need a Theme plugin.3.1 Installing dependencies
Add the plugin platform as a dependency to your application or plugin, either directly or transitively. To do so directly edit yourBuildConfig.groovy
to look something like this:... plugins { ... compile ':platform-ui:1.0.RC1' }You can run your code now and browse to
http://localhost:8080/<appname>/platform/ui
, a special page available only in the development environment. From there you can explore some of the plugin platform features, preview UI Sets and Themes etc.A default unstyled Theme and UI Set are included out of the box. They're not particularly useful although they do provide some proof of concept defaults, so we'll install another to show what's possible.Installing a theme for the sample application
Installing a theme requires you to introduce a plugin that containes one or more themes, or create your own theme in your app.Let's use the vanilla Twitter Bootstrap theme.Add the following to the "plugins" section of your BuildConfig.groovy:runtime ':bootstrap-theme:1.0.RC2'Alternatively you can run
grails install-plugin bootstrap-theme
.With that done, you'll have the bootrap-theme templates read to go, as well as a transitive dependency on bootstrap-ui
which provides the required UI Set templates and resources. This is how many Bootstrap based themes could be written easily to provide different layouts etc, while reusing the same underlying UI Set. It turns out that the UI Set is the biggest piece of work - Themes are easy to implement.We don't need to do anything to configure the default theme in the app to use the Bootstrap theme because it is the only non-default theme we have installed. Platform UI will use the first installed theme in alphabetical order if we have not told it otherwise.
3.2 Adapting views to Themes
So far nothing will have changed in your app. A theme has no effect unless your GSP specifies a theme layout to use. This is because Themes are built on top of Sitemesh layouts, and a Theme layout is really a Sitemesh layout.So we just need to create a home page GSP that uses the theme tags to present our content. Create a new file ingrails-app/views/index.gsp
or edit what is there, to contain this:<html> <head> <theme:layout name="home"/> <theme:title text="home.page.title"></theme:title> <head> <body> <theme:zone name="body"> <ui:h1 text="home.page.body.heading"/> <p><p:dummyText/></p> </theme:zone> <theme:zone name="banner"> <ui:carousel> <ui:slide active="${true}"> <ui:h1 text="Demo Banner Text"/> <ui:h2>Always says something interesting</ui:h2> </ui:slide> <ui:slide> <ui:h1 text="Inspiration"/> <ui:h2>Blue-skying maverick technologies</ui:h2> </ui:slide> <ui:slide> <ui:h1 text="Destabilizing"/> <ui:h2>Revolutionizing vertical potential</ui:h2> </ui:slide> </ui:carousel> </theme:zone> <theme:zone name="panel1"> <ui:h3 text="home.page.panel.1"/> <p><p:dummyText size="1"/></p> </theme:zone> <theme:zone name="panel2"> <ui:h3 text="home.page.panel.2"/> <p><p:dummyText size="1"/></p> </theme:zone> <theme:zone name="panel3"> <ui:h3 text="home.page.panel.3"/> <p><p:dummyText size="1"/></p> </theme:zone> </body> </html>Now run your app and browse to the home page. You should see something like this:
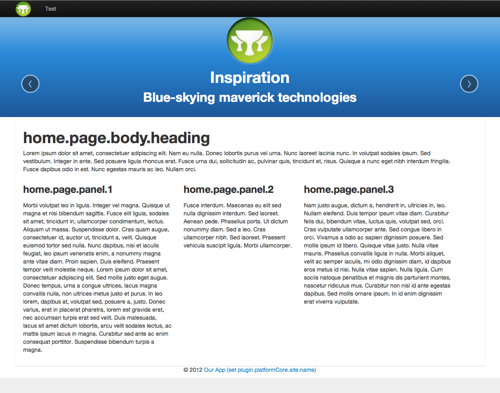
3.3 Creating forms with UI Sets
Creating a login form is a good example of how you can use Themes and UI Sets to build some UI that is independent of look and feel.Create aTestController
with a login
action and save login.gsp
in grails-app/views/test/
and place this in it:<html> <head> <theme:layout name="dialog"/> <theme:title text="login.page.title"></theme:title> <head> <body> <theme:zone name="body"> <ui:h1>Please log in</ui:h1> <ui:form> <ui:field name="username"/> <ui:field name="password" type="password"/> <ui:actions> <ui:button>Log in</ui:button> <ui:button>I forgot my password</ui:button> </ui:actions> </ui:form> </theme:zone> </body> </html>Now browse to
/test/login
and view the form. Notice how you have no styling or structure related code in your markup.If you had more themes installed you could switch to others to test the same form in them.